for문 처럼 배열의 반복을 하는 기능이다. 다만 index와 조건식을 따로 지정해 줄 필요가 없다.
기본 문법은
arr.forEach(callbackFunction(currentvalue, index, array), thisArg) 이다.
forEach의 매개변수로 callbackFunction과 thisArg가 있다. (thisArg는 선택적으로 사용)
callbackFunction의 매개변수로 value, index, array 가 있다. (index, array는 선택적으로 사용)
기본적인 사용방법은
var test = [{food : '아구찜', price : 35000},
{food : '족발', price : 25000},
{food : '닭발', price : 16000},
{food : '치느님', price: 18000}]
test.forEach(function(value, index, arr){
console.log('현재 돌고 있는 배열의 value : ', value)
console.log('현재 돌고 있는 배열의 index : ', index)
console.log('forEach에 사용되는 Array : ', arr)
})
결과
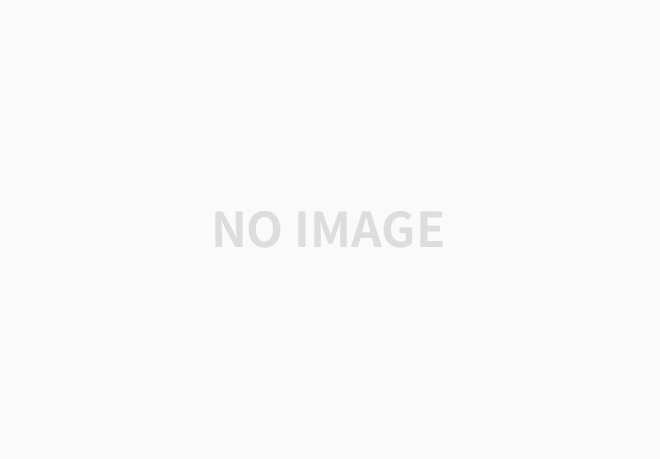
thisArg를 사용하는 경우.
thisArg 매개변수는 callbackFunction 내에서 this로 사용된다.
예를 들어 20000원 이하의 음식을 forEach를 이용해서 새로운 배열에 담을 때,
var test = [{food : '아구찜', price : 35000},
{food : '족발', price : 25000},
{food : '닭발', price : 16000},
{food : '치느님', price: 18000}]
var arg = {max : 20000}
var newArr = []
function testFunc(value){
if(value.price <= this.max){ // this가 arg를 대신해서 들어갔다.
newArr.push(value)
}
}
test.forEach(testFunc, arg)
console.log(newArr)
결과
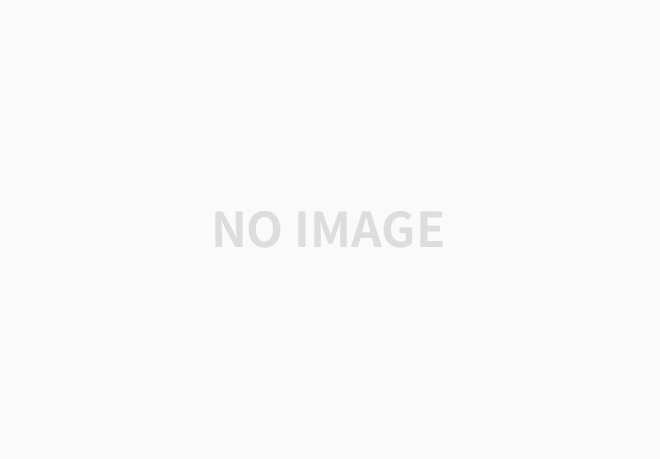
주의사항.
forEach는 throw 예외 처리를 해야 반복하는 도중에 중단시킬 수 있다.
조건을 만족할 때 까지만 반복을 해야한다면 기존의 for문을 이용해야 한다.
'개발 관련 지식 > JS' 카테고리의 다른 글
eslint (0) | 2020.03.07 |
---|---|
babel (0) | 2020.03.06 |
split : 문자열 분할 함수 (0) | 2020.03.05 |
filter 함수 (0) | 2020.03.03 |
문자열에서 특정 문자 찾기 (0) | 2020.03.01 |